Multi-agent network example¶
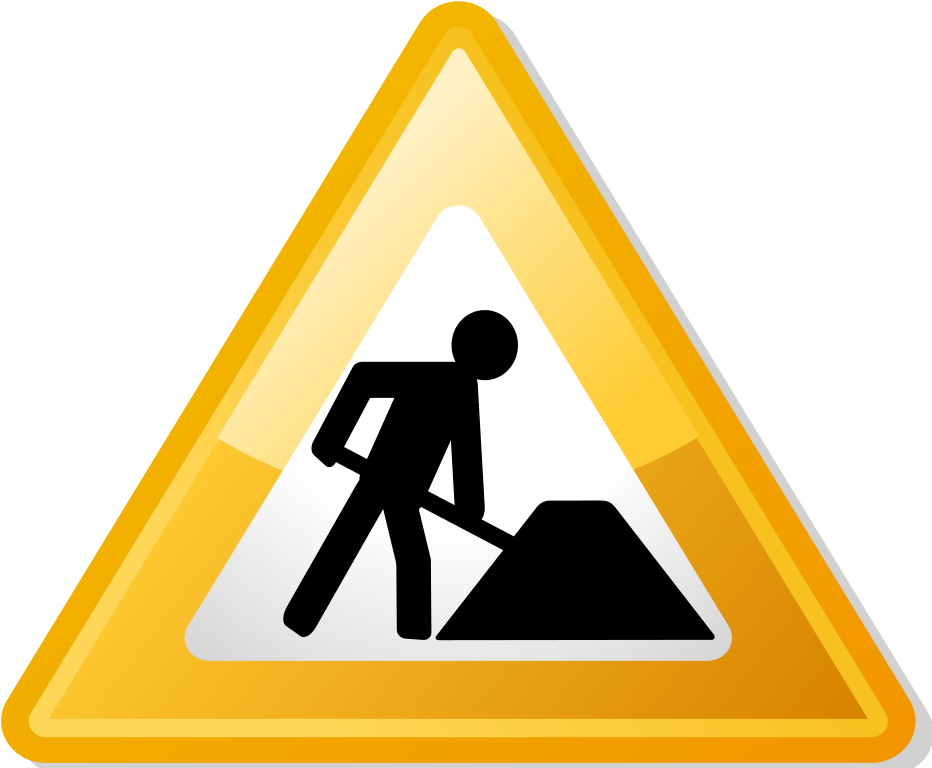
This page still under construction
This describes an experiment involving a coöperative binary choice game played by multiple players whose connectivity to one another varies in various ways, in an effort to understand how their networks of interconnectedness facilitate or hinders their abilities to succeed at the game.
paper1 [1],
paper2 [2],
paper3 [3].